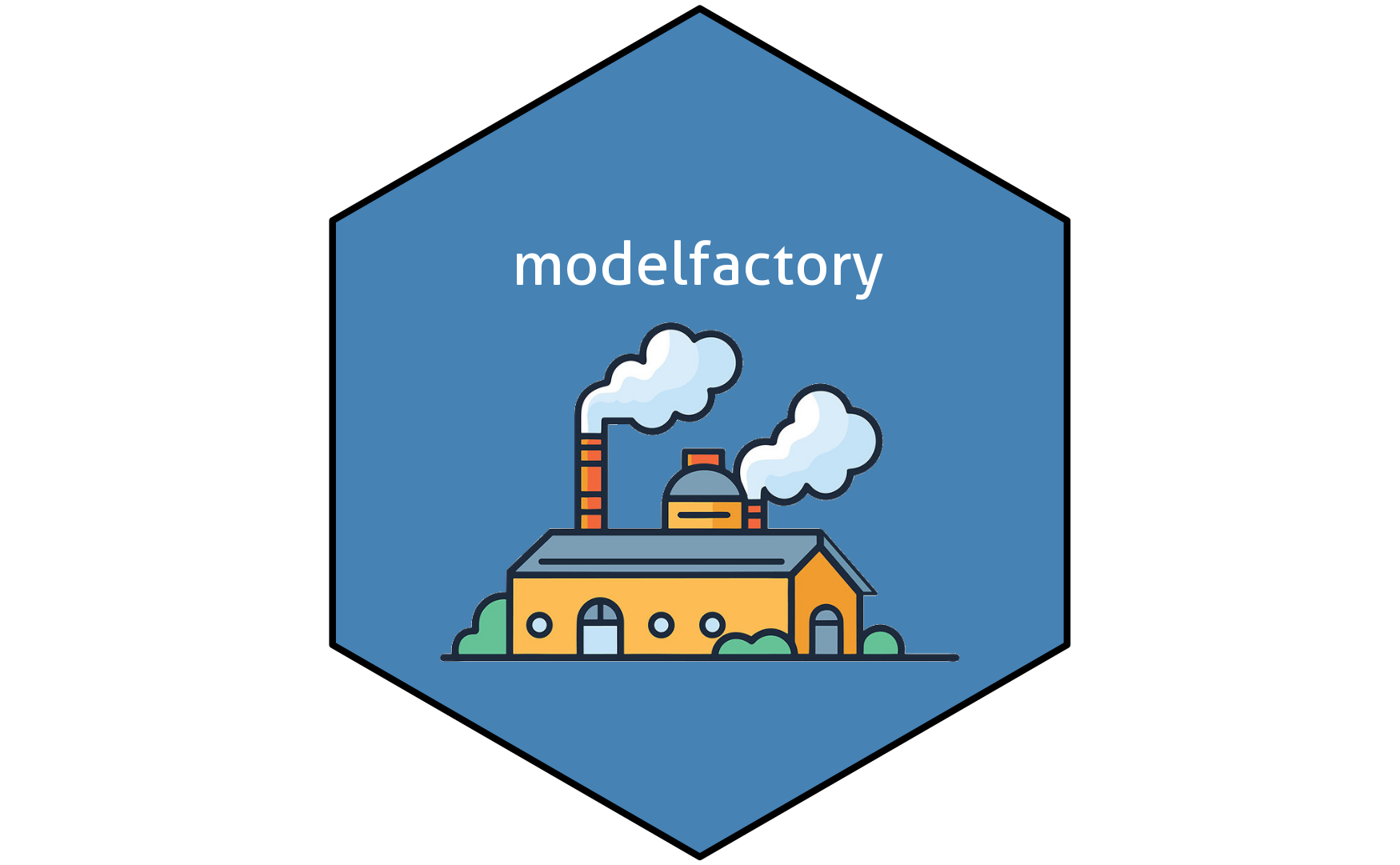
Stack coefficents, confidence intervals, and standard errors for n models.
Source:R/combine.R
stack_coeff.Rd
stack_coeff()
takes several lm or glm models, pulls out their coefficients,
standard errors, and confidence intervals, and stacks everything into a
tibble()
for easy comparison across models.
Examples
# multiple lm example ----------------------------------
lm_1 = lm(mpg ~ cyl + disp + hp, data = mtcars)
lm_2 = lm(mpg ~ hp + drat + wt, data = mtcars)
lm_3 = lm(mpg ~ ., data = mtcars)
lm_combined = stack_coeff(lm_1, lm_2, lm_3)
lm_combined
#> # A tibble: 19 × 7
#> coefficient model_name estimate std_error p_value lower_ci upper_ci
#> <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 (Intercept) mpg ~ cyl + disp +… 34.2 2.59 1.54e-13 28.9 39.5
#> 2 cyl mpg ~ cyl + disp +… -1.23 0.797 1.35e- 1 -2.86 0.406
#> 3 disp mpg ~ cyl + disp +… -0.0188 0.0104 8.09e- 2 -0.0401 0.00247
#> 4 hp mpg ~ cyl + disp +… -0.0147 0.0147 3.25e- 1 -0.0447 0.0153
#> 5 (Intercept) mpg ~ hp + drat + … 29.4 6.16 5.13e- 5 16.8 42.0
#> 6 hp mpg ~ hp + drat + … -0.0322 0.00892 1.18e- 3 -0.0505 -0.0139
#> 7 drat mpg ~ hp + drat + … 1.62 1.23 1.99e- 1 -0.898 4.13
#> 8 wt mpg ~ hp + drat + … -3.23 0.796 3.64e- 4 -4.86 -1.60
#> 9 (Intercept) mpg ~ . 12.3 18.7 5.18e- 1 -26.6 51.2
#> 10 cyl mpg ~ . -0.111 1.05 9.16e- 1 -2.28 2.06
#> 11 disp mpg ~ . 0.0133 0.0179 4.63e- 1 -0.0238 0.0505
#> 12 hp mpg ~ . -0.0215 0.0218 3.35e- 1 -0.0668 0.0238
#> 13 drat mpg ~ . 0.787 1.64 6.35e- 1 -2.61 4.19
#> 14 wt mpg ~ . -3.72 1.89 6.33e- 2 -7.65 0.224
#> 15 qsec mpg ~ . 0.821 0.731 2.74e- 1 -0.699 2.34
#> 16 vs mpg ~ . 0.318 2.10 8.81e- 1 -4.06 4.69
#> 17 am mpg ~ . 2.52 2.06 2.34e- 1 -1.76 6.80
#> 18 gear mpg ~ . 0.655 1.49 6.65e- 1 -2.45 3.76
#> 19 carb mpg ~ . -0.199 0.829 8.12e- 1 -1.92 1.52
# sometimes you might just want 1 model's summary ------
single_lm = stack_coeff(lm_1)
single_lm
#> # A tibble: 4 × 7
#> coefficient model_name estimate std_error p_value lower_ci upper_ci
#> <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 (Intercept) mpg ~ cyl + disp + … 34.2 2.59 1.54e-13 28.9 39.5
#> 2 cyl mpg ~ cyl + disp + … -1.23 0.797 1.35e- 1 -2.86 0.406
#> 3 disp mpg ~ cyl + disp + … -0.0188 0.0104 8.09e- 2 -0.0401 0.00247
#> 4 hp mpg ~ cyl + disp + … -0.0147 0.0147 3.25e- 1 -0.0447 0.0153
# glm example ------------------------------------------
glm_1 = glm(vs ~ drat + hp, data = mtcars)
glm_2 = glm(vs ~ wt + qsec, data = mtcars)
glm_3 = glm(vs ~ ., data = mtcars)
glm_combined = stack_coeff(glm_1, glm_2, glm_3)
#> Waiting for profiling to be done...
#> Waiting for profiling to be done...
#> Waiting for profiling to be done...
glm_combined
#> # A tibble: 17 × 7
#> coefficient model_name estimate std_error p_value lower_ci upper_ci
#> <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 (Intercept) vs ~ drat + hp 0.656 0.566 0.256 -0.454 1.77
#> 2 drat vs ~ drat + hp 0.137 0.133 0.312 -0.124 0.397
#> 3 hp vs ~ drat + hp -0.00484 0.00104 0.0000639 -0.00687 -0.00281
#> 4 (Intercept) vs ~ wt + qsec -2.20 0.537 0.000309 -3.25 -1.15
#> 5 wt vs ~ wt + qsec -0.226 0.0495 0.0000857 -0.323 -0.129
#> 6 qsec vs ~ wt + qsec 0.188 0.0271 0.000000121 0.135 0.242
#> 7 (Intercept) vs ~ . -0.864 1.95 0.662 -4.69 2.96
#> 8 mpg vs ~ . 0.00341 0.0226 0.881 -0.0409 0.0477
#> 9 cyl vs ~ . -0.159 0.103 0.137 -0.360 0.0424
#> 10 disp vs ~ . -0.000895 0.00186 0.636 -0.00455 0.00276
#> 11 hp vs ~ . 0.00289 0.00222 0.208 -0.00146 0.00724
#> 12 drat vs ~ . 0.0204 0.170 0.906 -0.313 0.354
#> 13 wt vs ~ . -0.0636 0.213 0.768 -0.481 0.354
#> 14 qsec vs ~ . 0.124 0.0731 0.105 -0.0195 0.267
#> 15 am vs ~ . -0.213 0.216 0.334 -0.636 0.209
#> 16 gear vs ~ . 0.0286 0.155 0.855 -0.276 0.333
#> 17 carb vs ~ . -0.0358 0.0857 0.680 -0.204 0.132